May 09, 21 · List list = new List();Sep 26, 15 · From Java 7 onwards, you can use a shorthand like Map foo = new HashMap();Your second way of instantiation is not recommended Stick to using List which is an Interface // Don't bind your Map to ArrayList new TreeMap();

Java Stack Stack In Java Journaldev
Java instantiate list
Java instantiate list-Java documentation Instantiating a generic type Example Due to type erasure the following will not work public void genericMethod() { T t = new T();Java ArrayList The ArrayList class is a resizable array, which can be found in the javautil package The difference between a builtin array and an ArrayList in Java, is that the size of an array cannot be modified (if you want to add or remove elements to/from an array, you have to create a new one) While elements can be added and removed from an ArrayList whenever you want



Understanding Observable Collections Collections And Concurrency Javafx 2 Part 1
Java by Faithful Finch on Aug 21 Donate Comment 1 ArrayList list=new ArrayList ();//Creating arraylist listadd ("Mango");//Adding object in arraylist listadd ("Apple");//This will add an item to the end of Linkedlist demollofferLast("Last_item_2");// Use List interface type instead new TreeMap();
// Create& initialize the list using unmodifiableList method List intlist = CollectionsunmodifiableList( ArraysasList(1,3,5,7));MyListadd ( "list" );//Printing the arraylist object Systemoutprintln (list);
Dec 11, 18 · Attention reader!Then today I read about using an anonymous subclass with instanceIf you want to declare a similar List of Strings in Groovy, you can use it's literal syntax




Java List How To Create Initialize Use List In Java




Java Cannot Instantiate The Type Configuration Mirror Api Stack Overflow
ArrayList is an implementation class of List interface in Java It is used to store elements It is based on a dynamic array concept that grows accordinglyJun 05, 13 · In Java, List is an interface That is, it cannot be instantiated directly Instead you can use ArrayList which is an implementation of that interface that uses an array as its backing store (hence the name) Since ArrayList is a kind of List, youPublic class Main { public static void main(String args) { // empty list List list = new ArrayList();
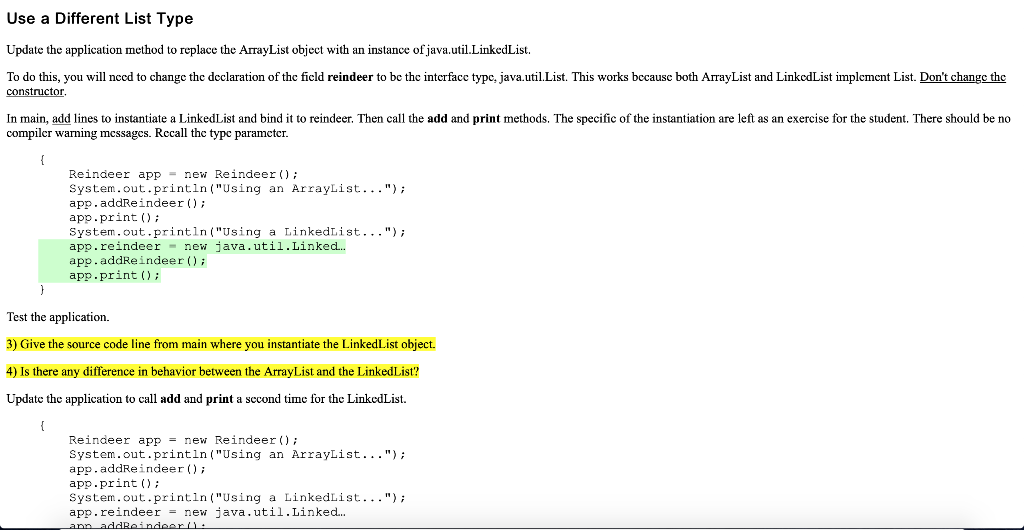



Please Answer Questions 1 To 5 That Is Highlighted Chegg Com
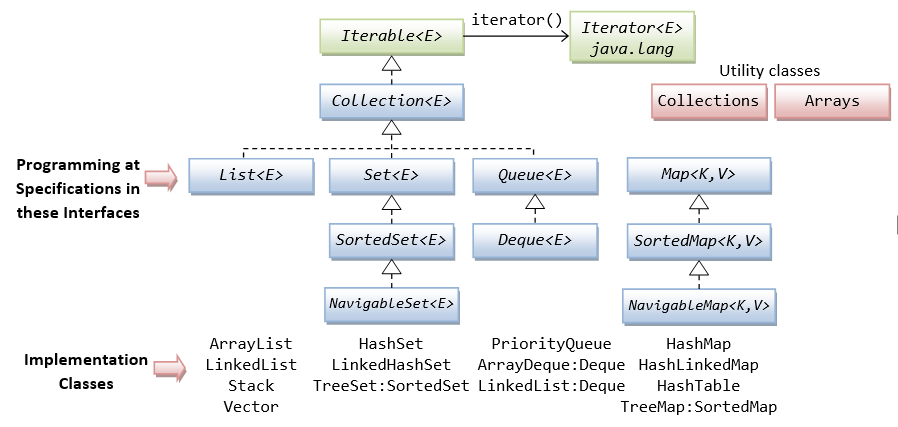



The Collection Framework Java Programming Tutorial
Resizablearray implementation of the List interface Implements all optional list operations, and permits all elements, including nullIn addition to implementing the List interface, this class provides methods to manipulate the size of the array that is used internally to store the list (This class is roughly equivalent to Vector, except that it is unsynchronized)Oct 08, 19 · ArraysasList () – Initialize arraylist from array To initialize an arraylist in single line statement, get all elements in form of array using ArraysasList method and pass the array argument to ArrayList constructor Create arraylist in single statement810 Code Practice with ArrayLists ¶ Fix the following code so that it compiles The code should instantiate an ArrayList of Strings names and fill it with the Strings from the array friends It should then print out names In line 2, capitalize the l in Arraylist so that the proper library is imported
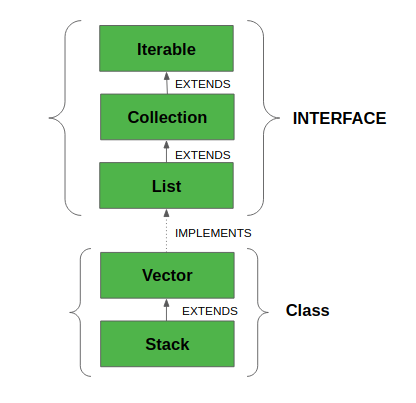



Stack Class In Java Geeksforgeeks
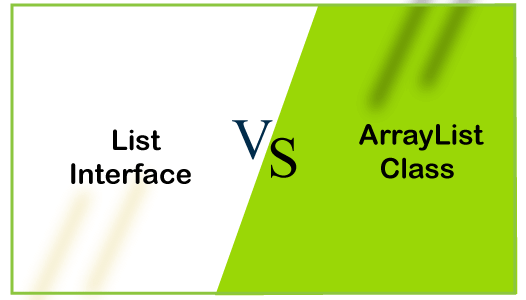



Difference Between List And Arraylist Javatpoint
It is found in the javautil package It is like the Vector in C The ArrayList in Java can have the duplicate elements also It implements the List interface so we can use all the methods of List interface here The ArrayList maintains the insertion order internally It inherits the AbstractList class and implements List interfaceInstead of instantiating an ArrayList directly,I am using a List to refer to ArrayList object so that we are using only the List interface methods and do not care about its actual implementationYou can use ArraysasList() method to create and initialize List at same line javautilArrays class act as bridge between Array and List in Java and by using this method you can quickly create a List from Array, which looks like creating and initializing List in one line, as shown in to instantiate a javautilProperties instance with values loaded from the from INFORMASJO TDT4105 at
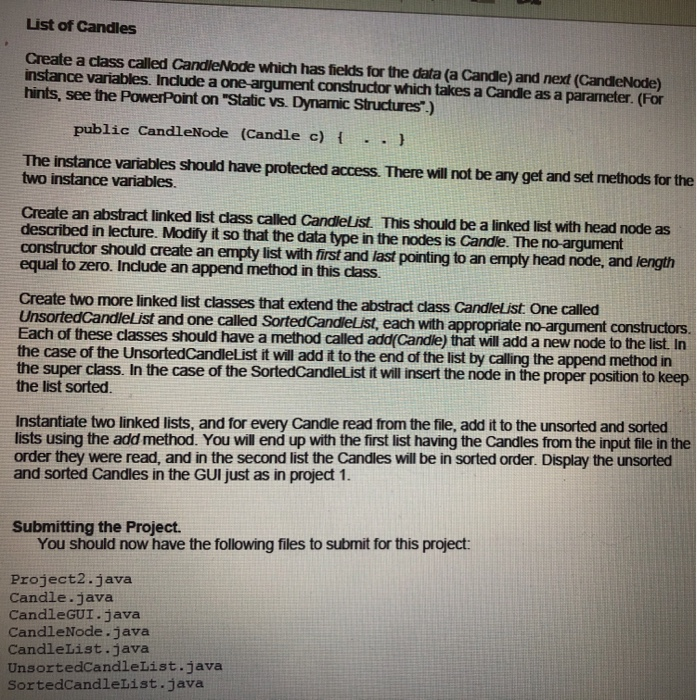



Solved List Candles Create Class Called Candlenode Fields Data Cande Next Candenode Instance Vari Q




Nested List In Java Code Example
List can be instantiated by any class implementing the interfaceBy this way,Java provides us polymorphic behaviourSee the example below List list = new ArrayList();Dec 11, 18 · Get hold of all the important Java Foundation and Collections concepts with the Fundamentals of Java and Java Collections Course at a studentfriendly price and become industry ready To complete your preparation from learning a language to DS Algo and many more, please refer Complete Interview Preparation CourseList list = new ArrayList();




Java List How To Create Initialize Use List In Java
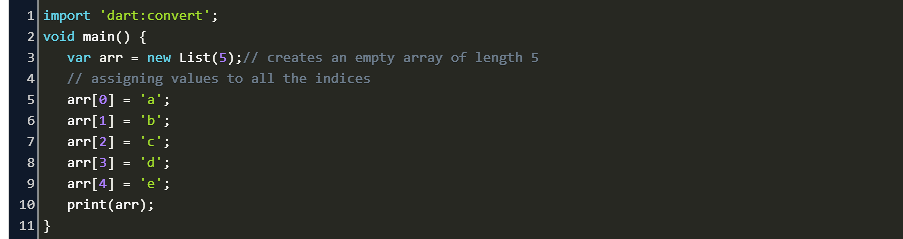



Declaring And Initializing A List In Dart Code Example
// Instantiating list using CollectionsaddAll() CollectionsaddAll(list, 10, , 30, 40);Feb 26, · Java Defining, Instantiating, and Starting Threads Last update on February 26 (UTC/GMT 8 hours) Introduction One of the most appealing features in Java is the support for easy thread programming Java provides builtin support for multithreaded programming A multithreaded program contains two or more parts that can runMar 01, 17 · List newlist = new ArrayList();




How To Iterate Through Linkedlist Instance In Java Crunchify
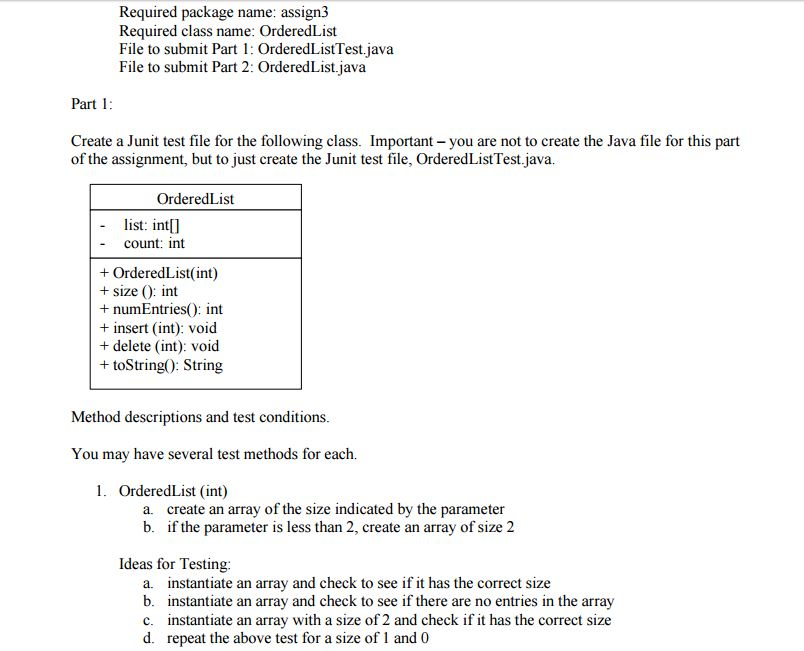



Answered Required Package Name Assign3 Required Class Name Orderedlist File To Submit Part 1 Orderedlist Test Java File Grand Paper Writers
Jan 05, 21 · Java Is abstract class instantiated here!You can't because List is an interface and it can not be instantiated with new List () You need to instantiate it with the class that implements the List interface Here are the common java Collections classes which implement List interfaceNov , 06 · Instantiating and populating a list or collection Posted on November , 06 by Todd Huss In Java it always irks me when I have to create a collection and then populate it in separate steps as follows List states = new ArrayList ();




Java Collections The List Interface Stack Abuse




Adding Value To List Initialize List Size Stack Overflow
List strings = new ArrayList ();// Print the listMyListadd ( "java" );




010 Java Programming Create A Class With Methods And Instantiate An Ob Java Programming Java Programming Tutorials Java




Java Ee How To Create A Concrete List Collection Class Arraylist And Linkedlist Using Util List In Spring
A quick and practical guide to picking a random item/items from a List in JavaThe List interface provides four methods for positional (indexed) access to list elements Lists (like Java arrays) are zero based Note that these operations may execute in time proportional to the index value for some implementations (the LinkedList class, for example) Thus, iterating over the elements in a list is typically preferable to1 Number of slices to send Optional 'thankyou' note Send No, you cannot instantiate enums, and there's a good reason for that Enums are for when you have a fixed set of related constants You don't want to instantiate one, because then the set would not be fixed If you really want to write your enum like the way you did, then you can



5 Marks In Java We Can Instantiate An Iterator Of A List Each Time The Iterator Is Called It Returns The Next Item In A List Implement A Course Hero




How To Implement A Linkedlist Class From Scratch In Java Crunchify
Nov 10, · November 10, January 29, 21 aminekouis 0 Comments declare and instantiate a number, declare vs initialize, initialize vs declare, initiate vs initialize, instantiate, instantiation and assignment, what do you mean by instantiation in java, what is the difference between instantiation and initialization of an object javaOct 07, · List strings = new ArrayList(ArraysasList( "Hello", "world" ));// Can not instantiate the type T }




Introduction To Linked Lists In C Dzone Web Dev
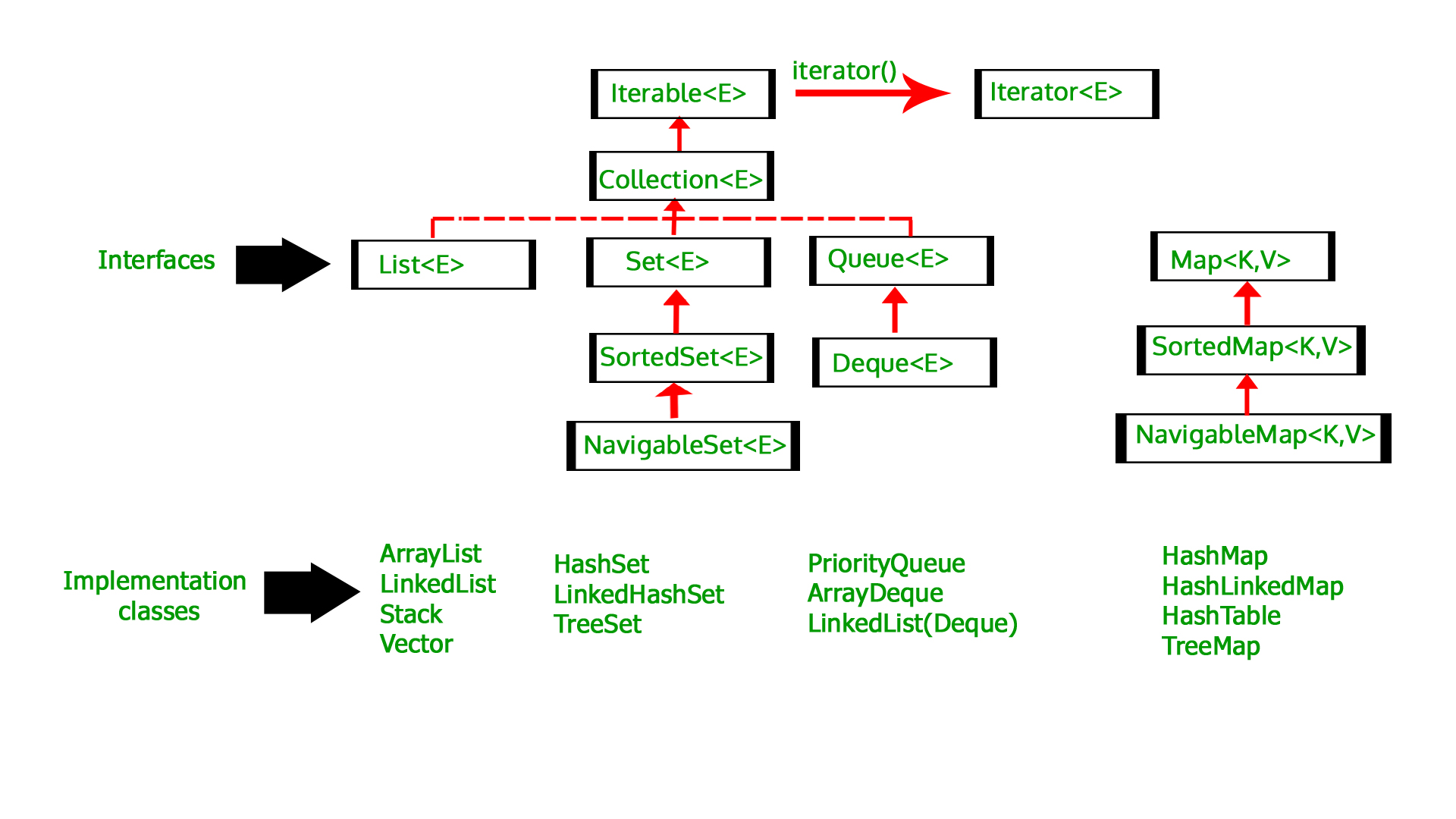



Initialize An Arraylist In Java Geeksforgeeks
I show my older approach below, but if you're using Java 7 or Java 8, this seems like a good approach My older approach This is the older, preJava 9 approach I used to use to create a static List in Java (ArrayList, LinkedList)Add Element to Collection Regardless of what Collection subtype you are using there are a few standard methods to add elements to a Collection Adding an element to a Collection is done via the add() methodJava initialize int list java one line list new arraylist in java with values string list initialization java initialize and populate list java initialize list of string java with values initialize list of string java instantiate a list java




Java67 How To Declare Arraylist With Values In Java Examples
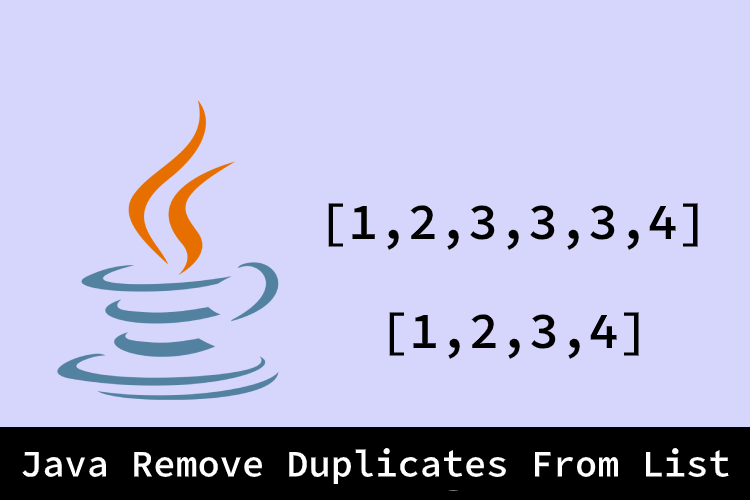



Java Remove Duplicates From List
MyListadd ( "a" );Yes, the answer is still the same, the abstract class can't be instantiated, here in the second example object of ClassOne is not created but the instance of an Anonymous Subclass of the abstract class And then you are invoking the method printSomething () on the abstractThat's all about how to declare an ArrayList with values in JavaYou can use this technique to declare an ArrayList of integers, String or any other object It's truly useful for testing and demo purpose, but I have also used this to create an ArrayList of an initial set of fixed values




How To Sort A Positional List In Java Study Com
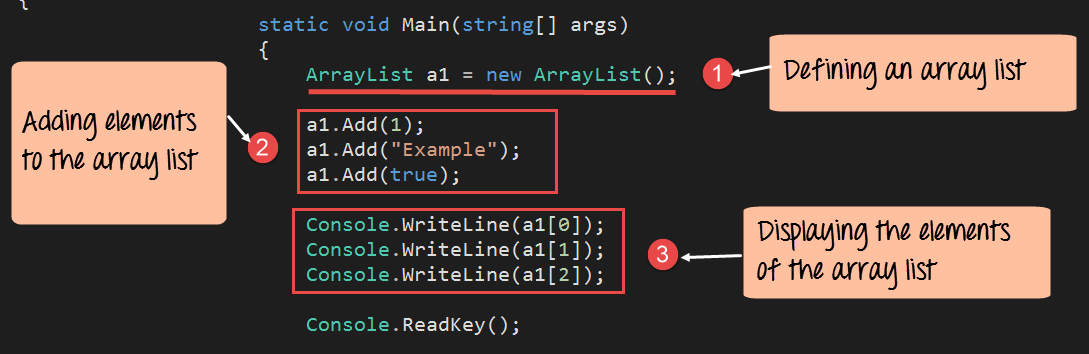



C Arraylist Tutorial With Examples
But that's not possible!In our post 3 ways to convert Array to ArrayList in Java, we have discussed about ArraysasList() method You can use ArraysasList() method to create and initialize List at same line javautilArrays class act as bridge between Array and List in Java and by using this method you can quickly create a List from Array, which looks like creating and initializing List in one line, asThis is somewhat incorrect;
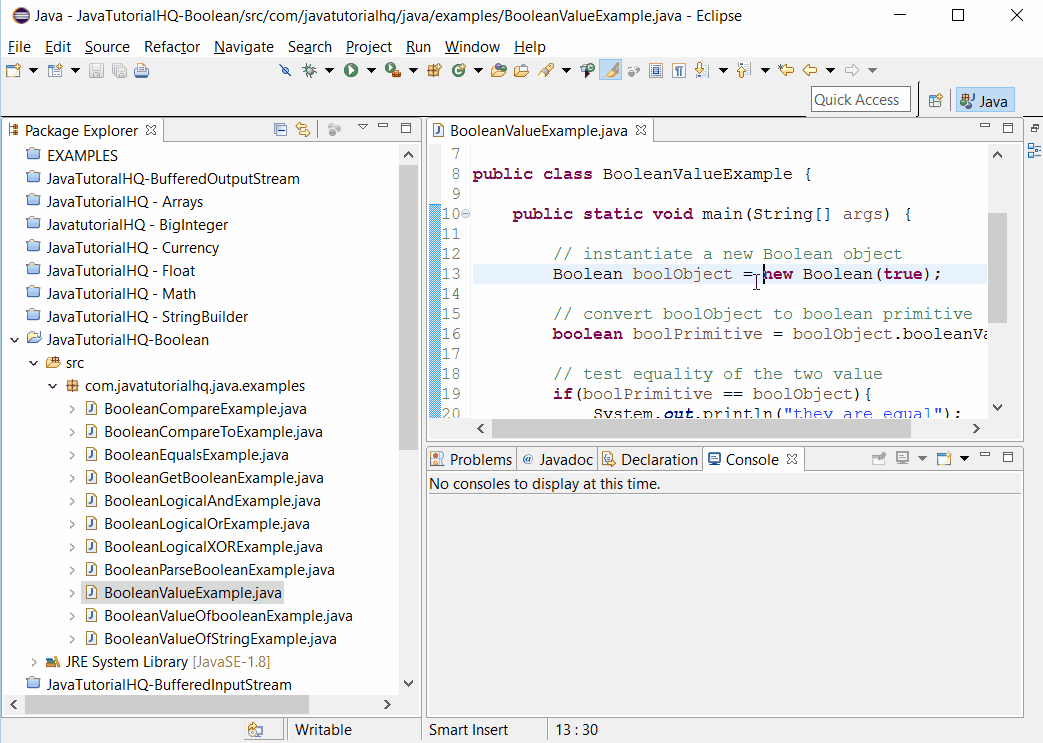



Java Boolean Booleanvalue Method Example
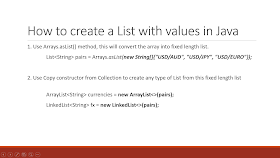



Javarevisited How To Declare And Initialize A List With Values In Java Arraylist Linkedlist Arrays Aslist Example
The method also has several alternatives, which set the range of an array to a particular valueThe Java List interface, javautilList, represents an ordered sequence of objectsThe elements contained in a Java List can be inserted, accessed, iterated and removed according to the order in which they appear internally in the Java ListThe ordering of the elements is why this data structure is called a List Each element in a Java List has an index// Print the list Systemoutprintln("List with addAll() " listtoString());




Xyz Code Declare Instantiate Initialize And Use A One Dimensional Array




Chapter 11 Array Based Lists Ppt Download
//This will add anOct 28, 17 · The javautilArrays class has several methods named fill(), which accept different types of arguments and fill the whole array with the same value long array = new long5;To instantiate an object in Java, follow these seven steps Open your text editor and create a new file Type in the following Java statements The object you



What Is Iterable Interface What Is Java Online Club Facebook



Understanding Observable Collections Collections And Concurrency Javafx 2 Part 1
A List is an interface, not an abstract class List is part of the Java Collection Framework, and so one can follow the trail all the way back to the Collection interface, which gives basic guidelines (creates a contract, so to speak, with the implementing class) on methods for storing objectsOct 05, 18 · Java ArrayList allows us to randomly access the list ArrayList can not be used for primitive types, like int, char, etc We need a wrapper class for such cases (see this for details) ArrayList in Java can be seen as similar to vector in C Below are the various methods to initialize an ArrayList in Java Initialization with add() SyntaxJan 13, 08 · In Java, instantiation of a List and filling it with some Strings are typically separated in multiple steps List myList = new ArrayList ();




Arraylist Subclass Programmer Sought
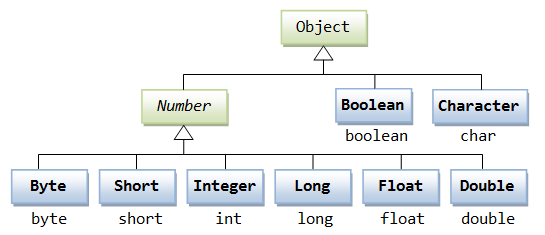



Java Programming Tutorial On Generics
Dec 03, 13 · Books stored in array list are Java Book1, Java Book2, Java Book3 Method 4 Use Collectionsncopies Collectionsncopies method can be used when we need to initialize the ArrayList with the same value for all of its elements Syntax count is number of elements and element is the item valueMar 14, 19 · Set set = new HashSet();Don't stop learning now Get hold of all the important Java Foundation and Collections concepts with the Fundamentals of Java and Java Collections Course at a studentfriendly price and become industry ready To complete your preparation from learning a language to DS Algo and many more, please refer Complete Interview Preparation Course



Cannot Instantiate Remote Class Issue 49 Oracle Visualvm Github




Java Stack Stack In Java Journaldev
This Tutorial Explains How to Declare, Initialize & Print Java ArrayList with Code Examples You will also learn about 2D Arraylist & Implementation of ArrayList in Java Java Collections Framework and the List interface were explained in detail in our previous tutorialsWhen you create an object, you are creating an "instance" of a class, therefore "instantiating" a class The new operator requires a single, postfix argument a call to a constructor The name of the constructor provides the name of the class to instantiate The new operator returns a reference to the object it createdMay 15, 17 · Java Instantiation Review To instantiate is to create an object from a class using the new keyword From one class we can create many instances A class contains the name, variables and the




Cannot Find Symbol Symbol Class Simpledate Location Class Simpledateclient Stack Overflow



Org Hibernate Instantiationexception Cannot Instantiate Abstract Class Or Interface Java Util List
Failed to instantiate javautilList Specified class is an interface in GET request In my spring boot REST API application, I need to handle HTTP GET by accepting a stronglytyped list as my input @RestController public class CusttableController { @RequestMapping (value="/custtable", method=RequestMethodGET) public ListApr 24, · Create an immutable List from an ArrayList with the JDK, Guava or Apache Commons Collections Start Here;Question on list of lists In this posts, we will see how to create a list of lists in java You can easily create a list of lists using below syntax List listOfLists = new ArrayList ();
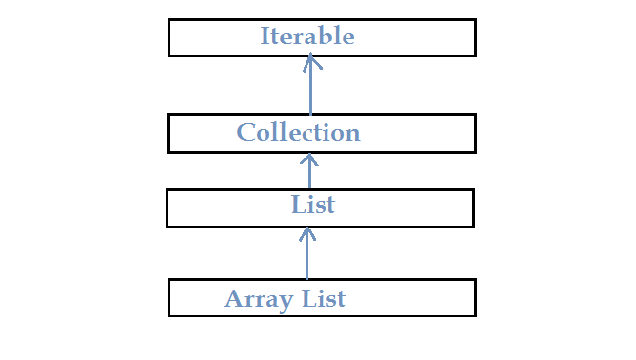



How To Create An Arraylist In Java Dzone Java




How To Implement A Linkedlist Class From Scratch In Java Crunchify
Oct 14, 19 · Therefore, to convert a LinkedList to an array − Instantiate the LinkedList class Populate it using the add () method Invoke the toArray () method on the above created linked list and retrieve the object array Convert each and every element of the object array to string
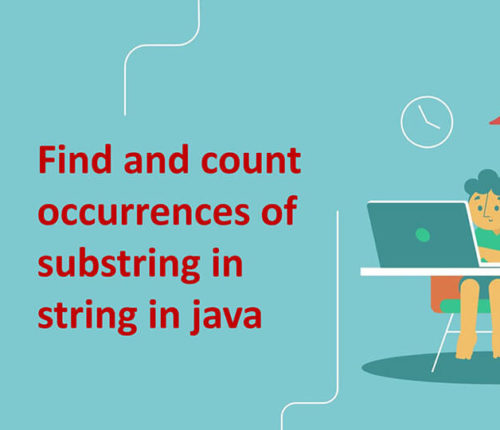



Initialize List Of String In Java Java2blog



Solved List Of Words Create A Class Called Wordnode Which Has Fields For The Data A Word And Next Wordnode Instance Variables Include A One Ar Course Hero



Cannot Instantiate The Type Java




How To Instantiate An Object In Java Webucator




Tutorials Page 15 Camposha




Java67 How To Initialize Hashmap With Values In Java One Liner
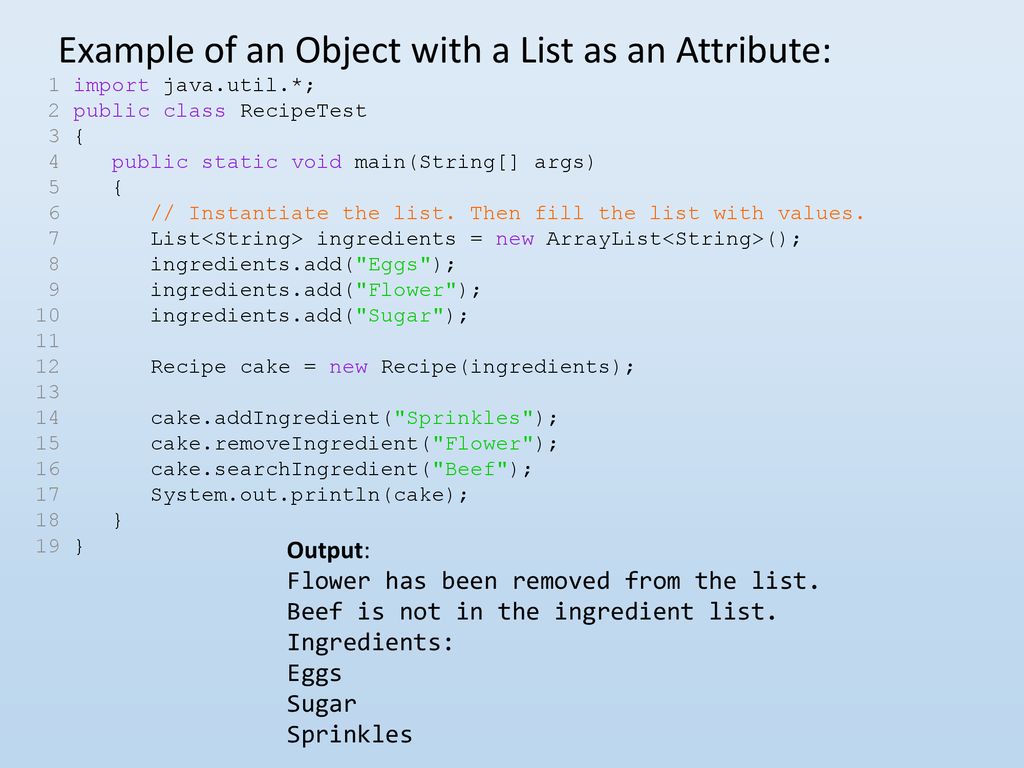



Objects With Arraylists As Attributes Ppt Download
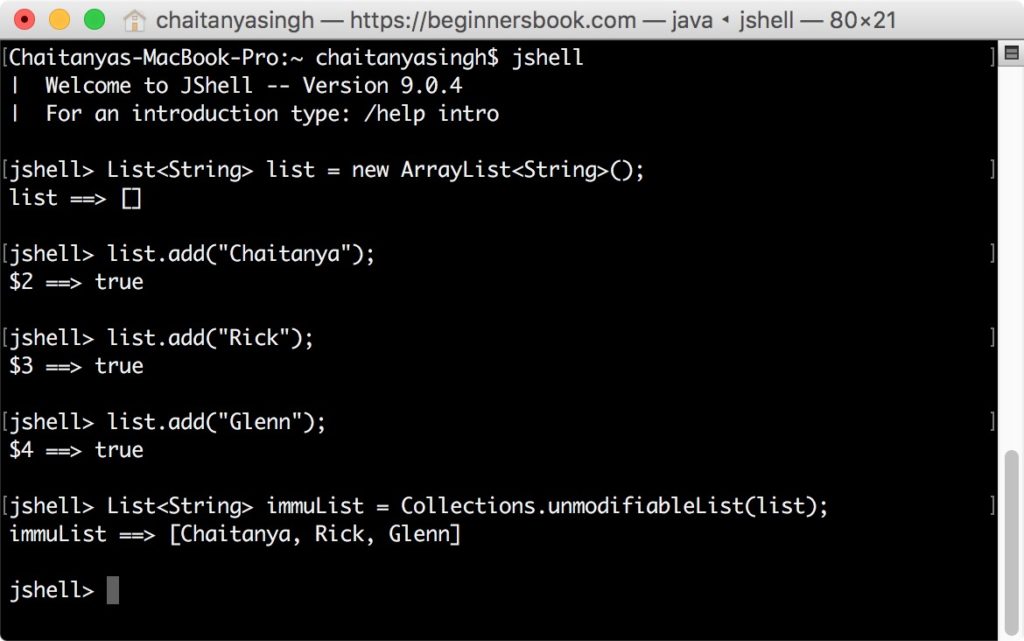



Java 9 Factory Method To Create Immutable List



Instantiating And Manipulating Linkedlist In Java With Examples




Java Optimization Casting Or Instantiating New Object Stack Overflow



Understanding Observable Collections Collections And Concurrency Javafx 2 Part 1
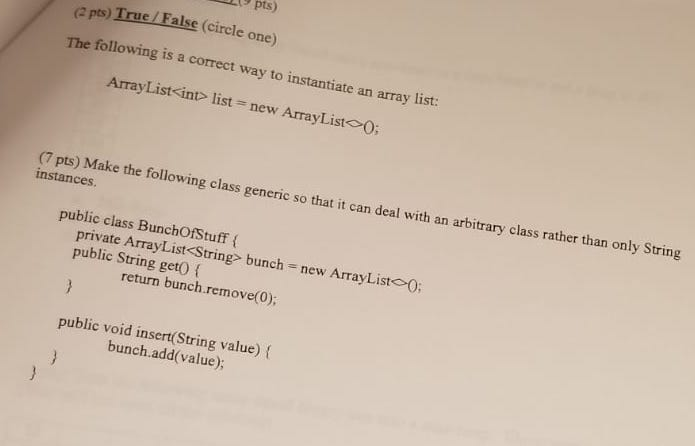



Solved Pts E Pts True False Circle One Following Correct Way Instantiate Array List Arraylist Lis Q
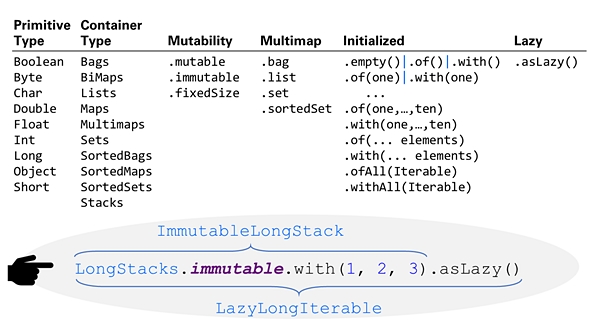



Refactoring To Eclipse Collections Making Your Java Streams Leaner Meaner And Cleaner




Parametric Polymorphism And Java Generics Ppt Download
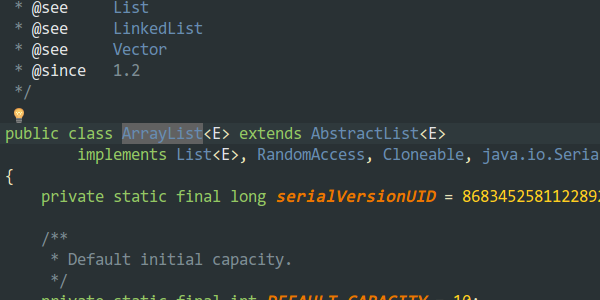



Java Arraylist Octoperf



Understanding Observable Collections Collections And Concurrency Javafx 2 Part 1
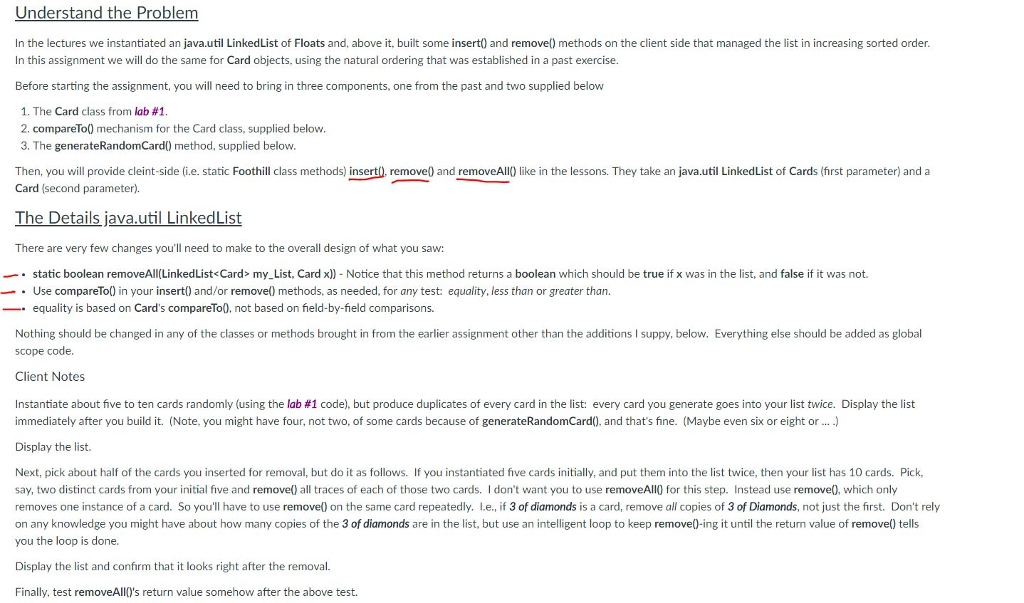



Solved Hello I Have An Assignment Which I Don T Know How Chegg Com




Reflection Page 2 Developers Corner Java Web Development Tutorials




The Java Background Interface Is List Lt String Gt Error Java Util List Specified Class Is An Interface Programmer Sought
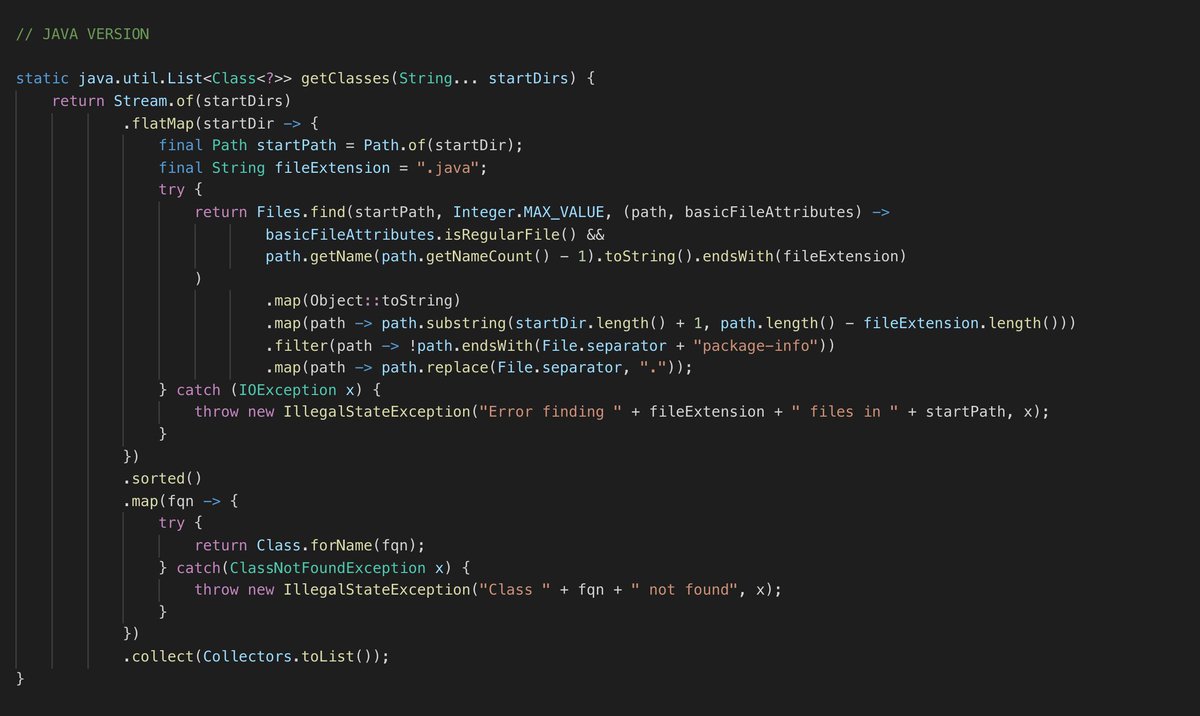



Daniel Dietrich Java Vs Vavr Example Search Directories For Java Files And Instantiate Their Corresponding Classes Both Implementations Are Basically Identical However It Shows That It Is Cumbersome To Wrap




How To Instantiate An Object In Java Webucator




Java List How To Create Initialize Use List In Java
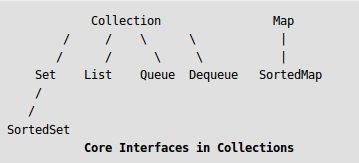



Initializing A List In Java Geeksforgeeks
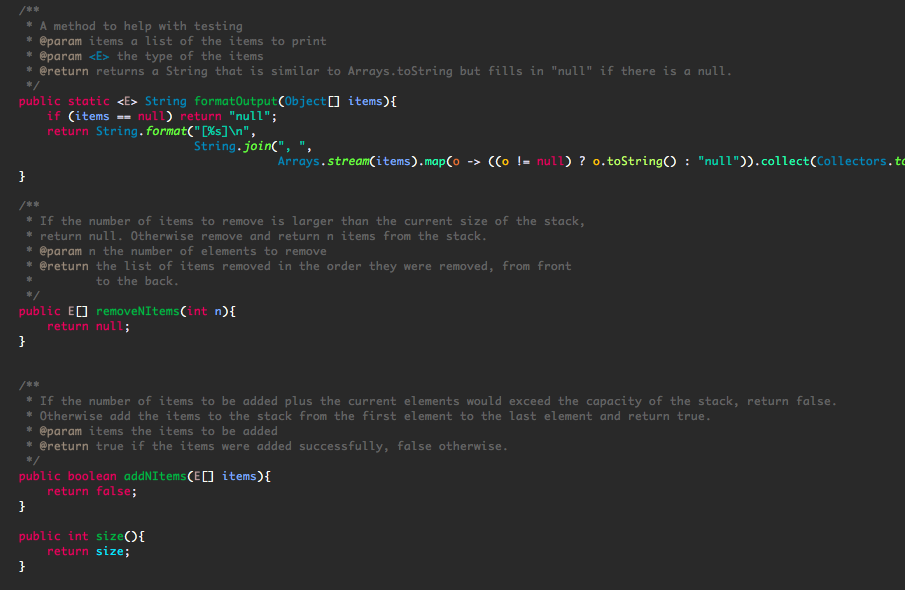



Import Java Util Arrays Chegg Com
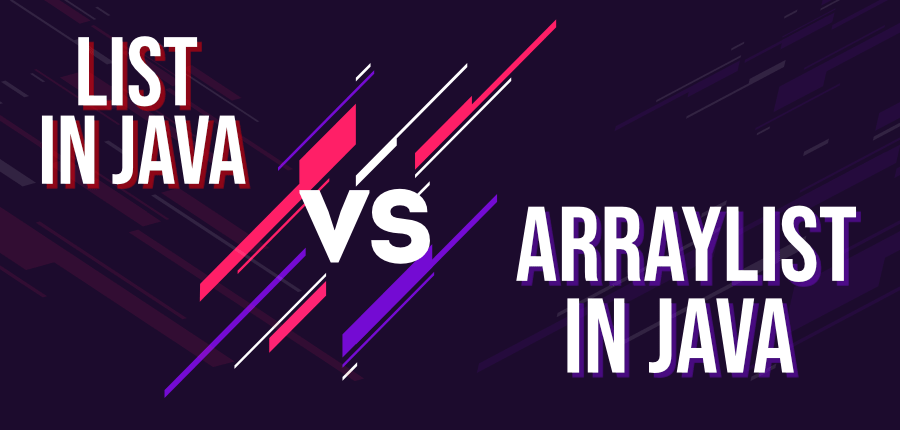



Difference Between List And Arraylist In Java Geeksforgeeks
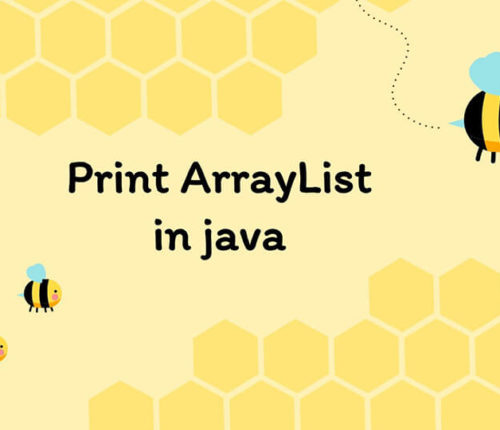



How To Create List Of Lists In Java Java2blog
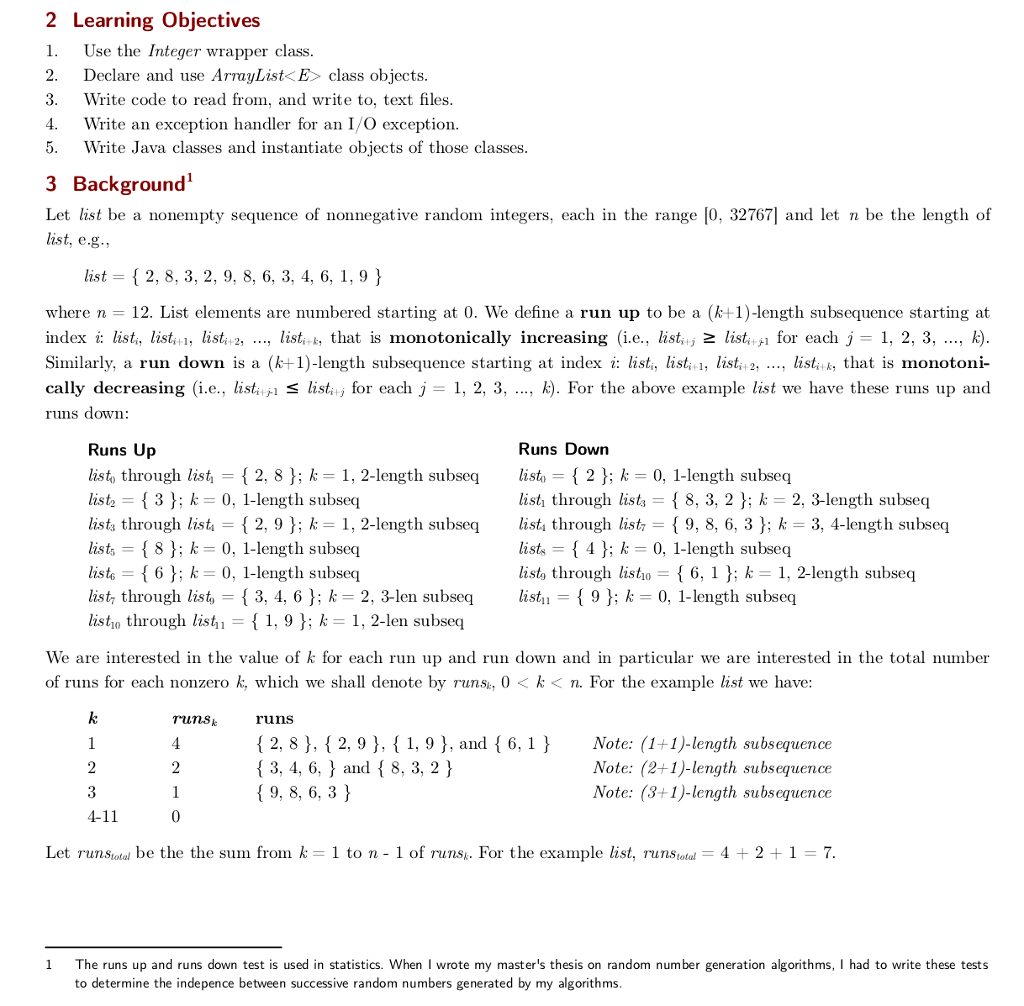



2 Learning Objectives 1 Use The Integer Wrapper Chegg Com



List Of Words Create A Class Called Wordnode Which Has Fields For The Data A Word And Next Wordnode Instance Variables Include A One Argument Course Hero
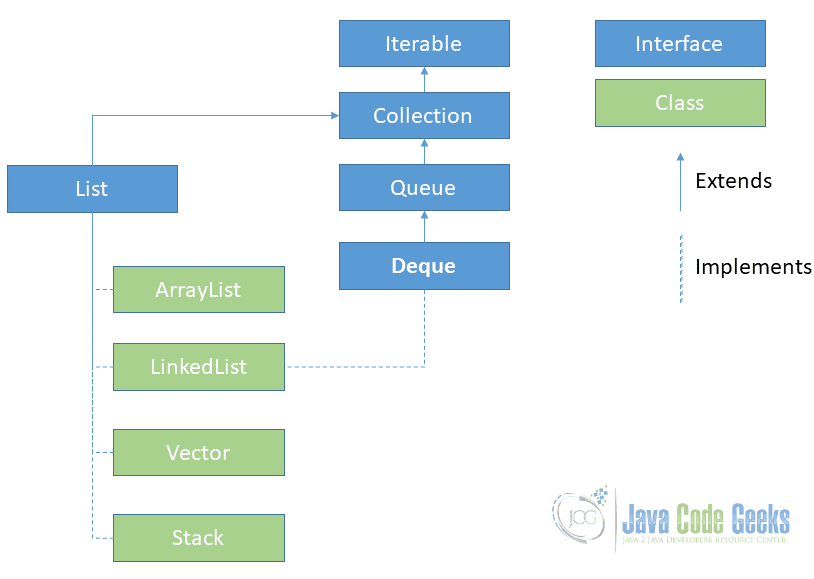



Java List Example Examples Java Code Geeks 21




Javarevisited How To Declare And Initialize A List With Values In Java Arraylist Linkedlist Arrays Aslist Example




Java Chapter 12 Collection Classes




Java Collections Framework Wikipedia
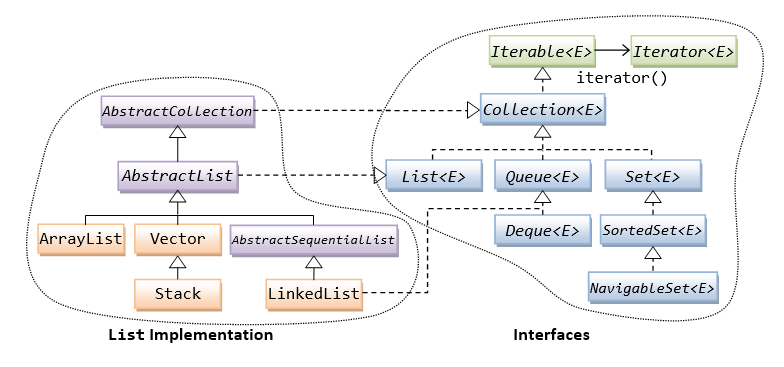



The Collection Framework Java Programming Tutorial




Cannot Instantiate The Type Webdriver Stack Overflow




Using Java Lists And Maps Collections Tutorial




Tip Java Instantiating Generic Type Parameter Geekycoder




Java List How To Create Initialize Use List In Java
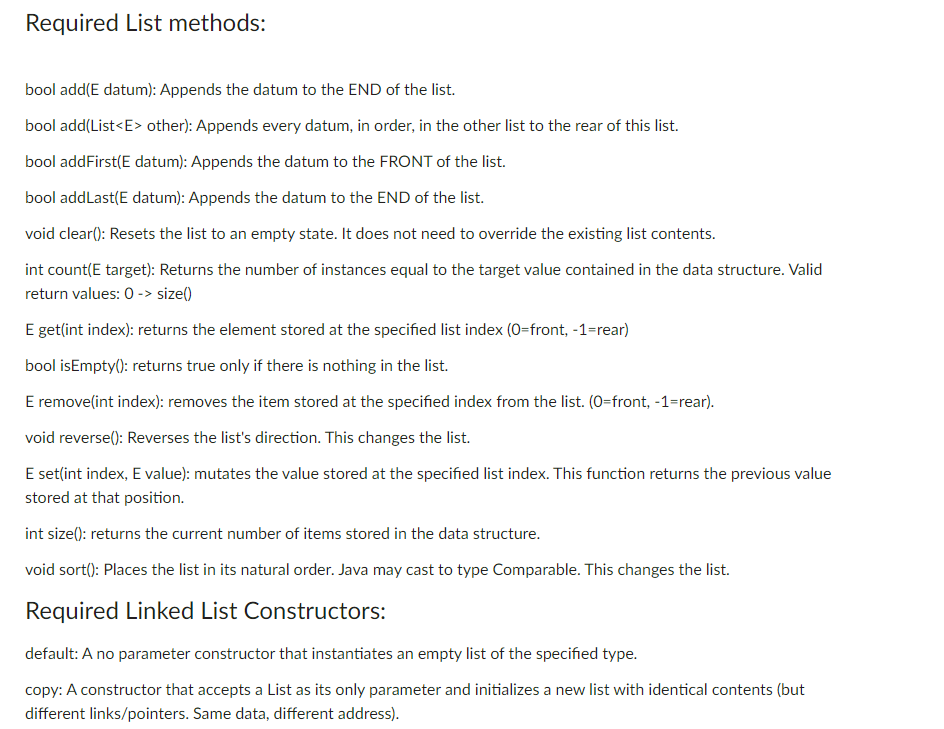



Using Either Java 1 8 Or C 11 Students Shall Chegg Com
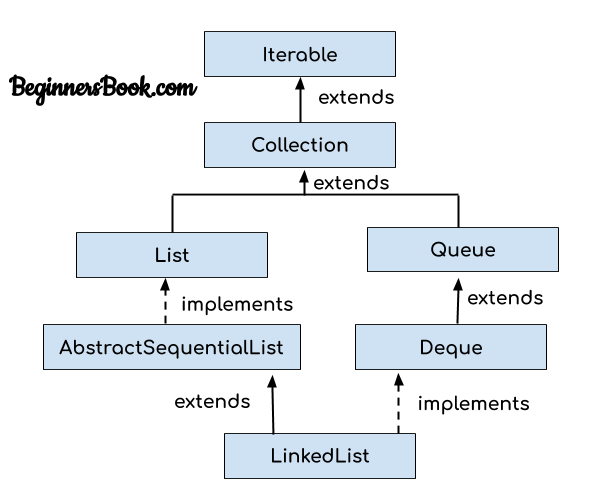



Linkedlist In Java With Example




How Do I Instantiate A Queue Object In Java Stack Overflow
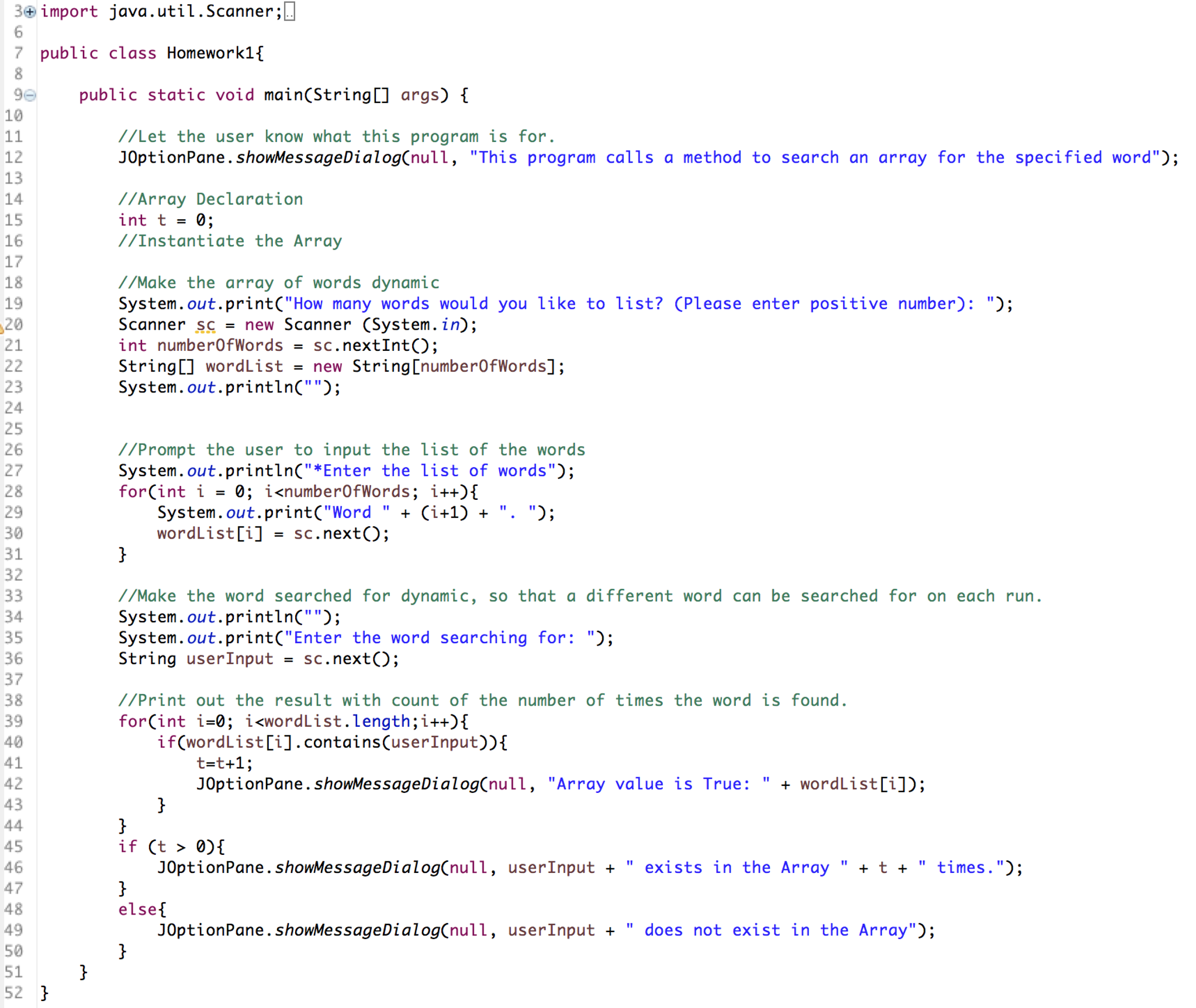



Solved Import Java Util Scanner Public Class Homeworkl P Chegg Com




Solve The Problem Of Failed To Instantiate Java Util List Specified Class Is An Interface Programmer Sought




Java Arraylist How To Declare Initialize Print An Arraylist




Java List How To Create Initialize Use List In Java
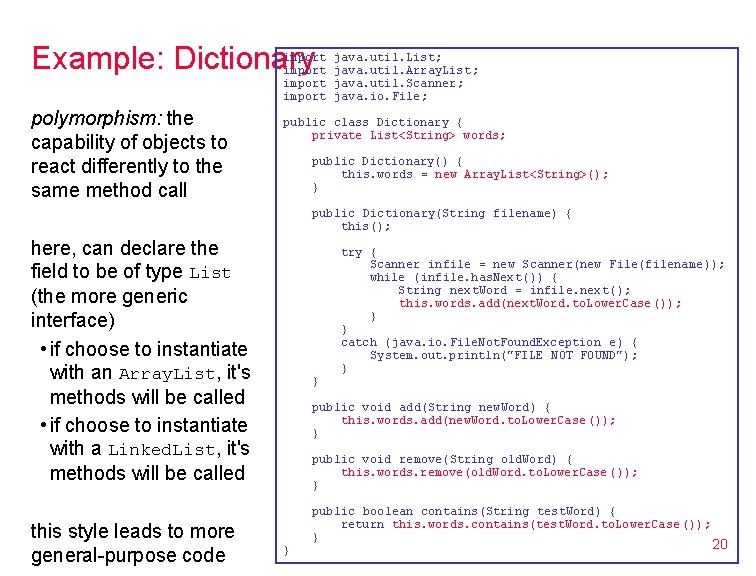



Csc 427 Data Structures And Algorithm Analysis Fall




Make An Empty List Java Code Example
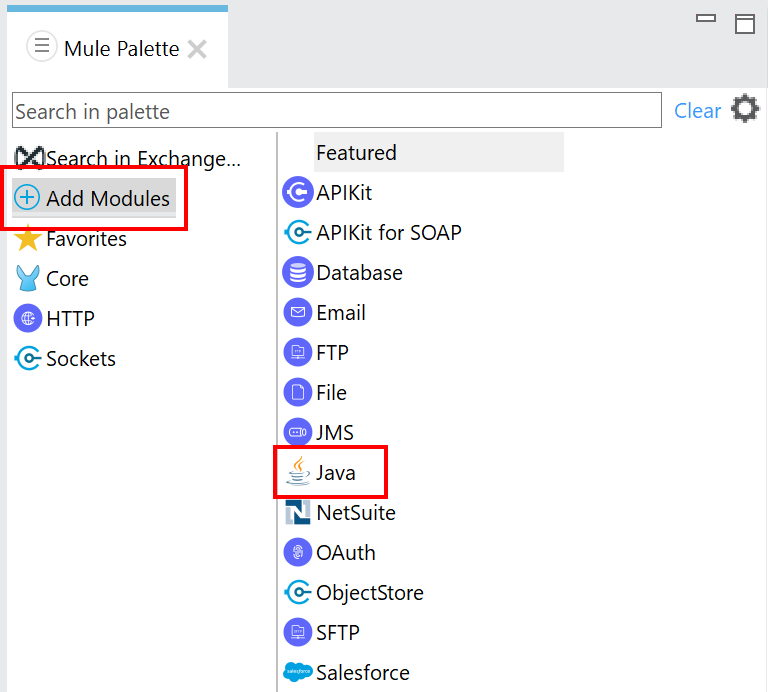



Java In Mule For Java Programmers Dzone Java




Adding Value To List Initialize List Size Stack Overflow
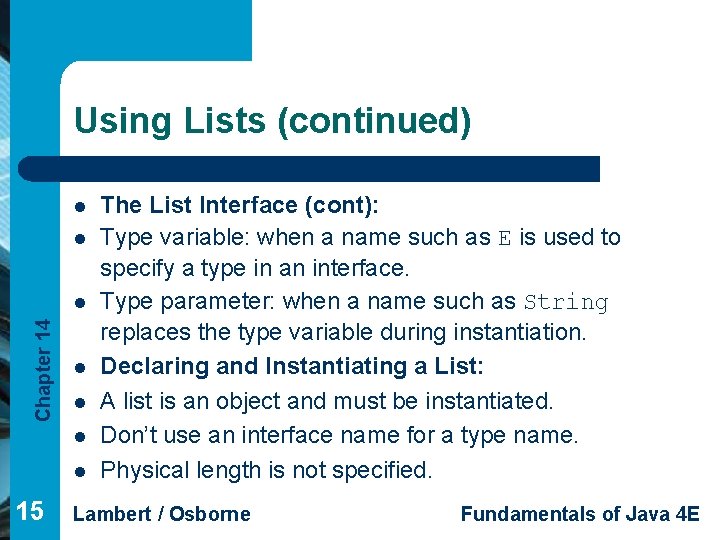



Chapter 14 Introduction To Collections Fundamentals Of Java




Java Array Of Arraylist Arraylist Of Array Journaldev
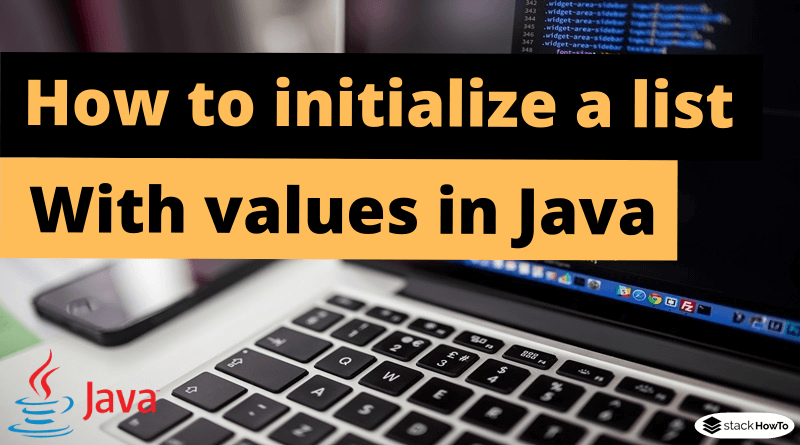



How To Initialize A List With Values In Java Stackhowto




Java Array Of Arraylist Arraylist Of Array Journaldev




Create A List Of Integers Containing 1 2 3 4 And 5 Java Code Example




Create A List In Java Code Example




Top 10 Mistakes Java Developers Make




Pin On Java
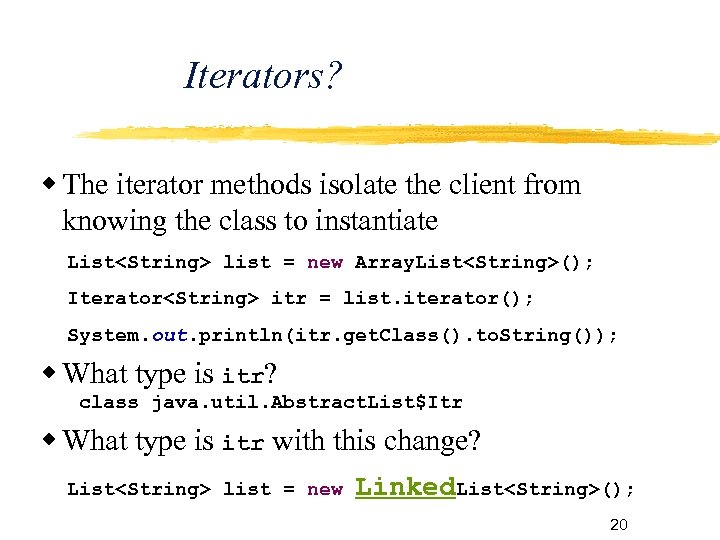



Creational Design Patterns Csc 335 Object Oriented Programming And



Java Set
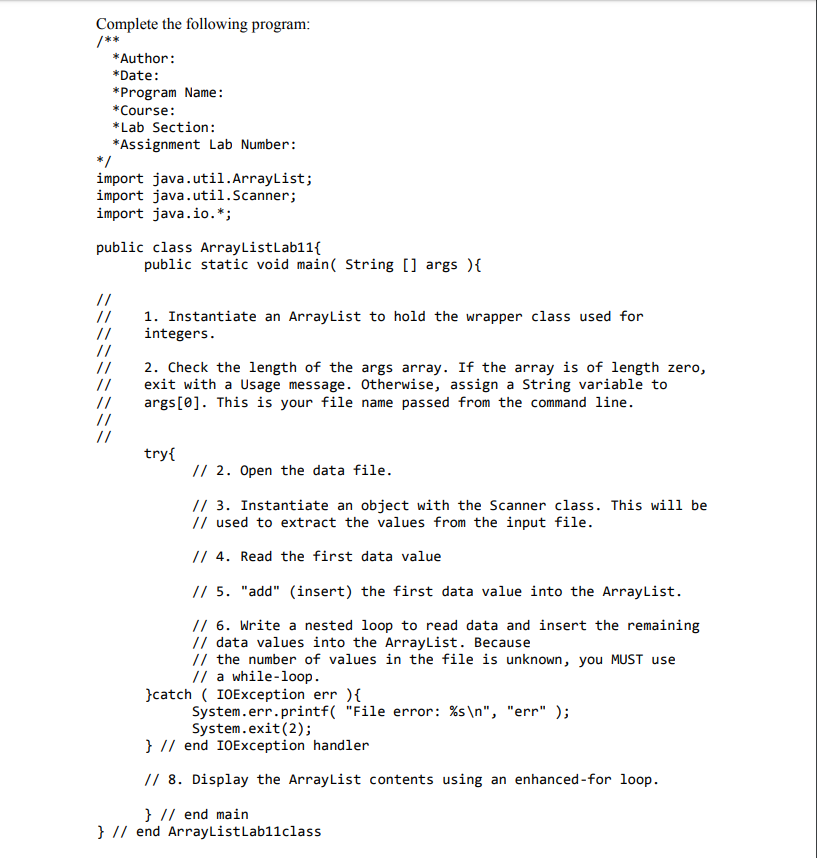



Solved Java Purpose Assignment Use Wrapper Class Arraylist Class Read List Random Numbers Contain Q




Chapter 12 The Arraylist Data Structure Section 1 How To Instantiate An Arraylist Section 2 The Arraylist Subset Section 3 Declaring An Arraylist Ppt Download




Collections In Java Javatpoint
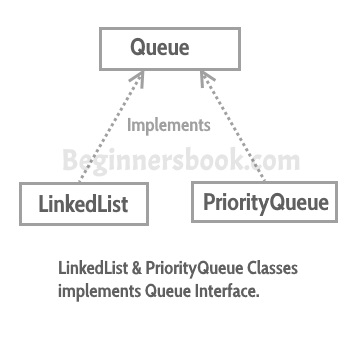



Queue Interface In Java Collections
0 件のコメント:
コメントを投稿